LLM Agents Transforming Code Generation Workflows
The landscape of software development is evolving rapidly, largely driven by advancements in artificial intelligence (AI) and machine learning (ML). Among the most transformative technologies are Large Language Models (LLMs) that can understand, generate, and manipulate code. LLMs have emerged as powerful tools in programming assistance and code generation, significantly enhancing productivity and creativity in software development.
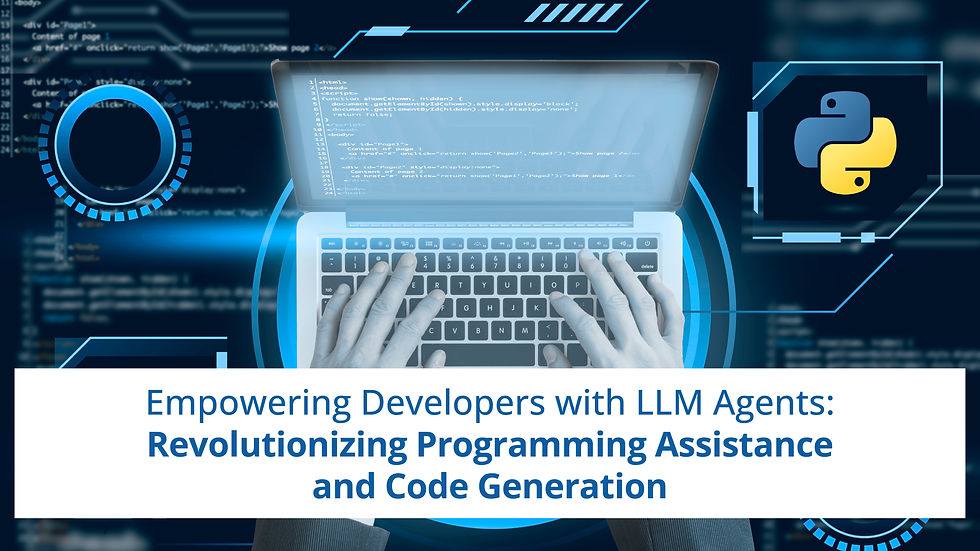
This article explores the diverse use cases of LLM agents in these domains, showcasing how they can revolutionize the way developers write and maintain code.
Intelligent Code Suggestions
One of the most prominent use cases of LLMs in programming assistance is their ability to provide intelligent code suggestions. These models analyze existing code and understand the context to offer recommendations for code snippets, functions, or even entire modules. This feature is particularly beneficial for both novice and experienced developers who may struggle with syntax or logic errors.
For instance, when a developer is writing a function to sort an array, an LLM can suggest the most efficient sorting algorithm based on the data type and context. This not only saves time but also helps improve code quality by promoting best practices and efficient solutions.
Example:
Consider a scenario where a developer types out a function header in Python:
python
def sort_array(arr):
An LLM can intelligently complete the function body with a recommended sorting algorithm, such as:
python
def sort_array(arr):
return sorted(arr)
By offering such suggestions, LLMs empower developers to write cleaner, more efficient code while minimizing the risk of errors.
Code Documentation Generation
Documentation is a critical aspect of software development that often gets overlooked due to time constraints. LLMs can automate the generation of code documentation by analyzing code and creating comments that explain the functionality of various code segments.
This use case is especially useful in large codebases where maintaining up-to-date documentation is a challenge. By generating comments and docstrings automatically, LLMs enable developers to focus on coding rather than documenting, ensuring that the code is always accompanied by clear explanations.
Example:
A developer writes the following function:
python
def calculate_area(radius):
return 3.14 radius * 2
An LLM can generate the documentation as follows:
python
def calculate_area(radius):
"""Determine the area of a circle based on its radius."""
return 3.14 radius * 2
This automatic documentation not only improves code readability but also enhances the overall quality of the software.
Debugging and Error Resolution
Debugging is often one of the most tedious and time-consuming aspects of programming. LLMs can assist developers in identifying and resolving errors by analyzing the code and suggesting potential fixes. This capability can significantly reduce the time spent on debugging and enhance the overall development process.
When a developer encounters an error message, an LLM can provide insights into the possible causes of the issue and recommend corrective actions. This can be particularly valuable for new developers who may lack experience in debugging complex code.
Example:
Imagine a developer receives an error related to a missing variable:
python
print(variable_not_defined)
An LLM can analyze the context and suggest possible definitions or checks:
python
# Verify whether the variable has been initialized.
if 'variable_not_defined' not in locals():
variable_not_defined = 0 # Assign a default value
By offering such solutions, LLMs can help developers learn debugging techniques and improve their problem-solving skills.
Code Refactoring
Refactoring code is an essential practice that enhances readability, maintainability, and performance. LLMs can assist developers in identifying areas of code that require refactoring and provide suggestions to improve structure and clarity. This not only results in cleaner code but also promotes best practices in software design.
For example, if a developer writes repetitive code, an LLM can suggest creating a function to encapsulate that logic, thereby reducing duplication and improving maintainability.
Example:
Consider a scenario where a developer has the following repeated code:
python
def process_data1(data):
# Data processing logic here
def process_data2(data):
# Data processing logic here
An LLM could suggest:
python
def process_data(data):
# Data processing logic here
def process_data1(data):
process_data(data)
def process_data2(data):
process_data(data)
This refactoring not only reduces code duplication but also makes future updates easier.
Code Translation
With the increasing demand for multi-language software, code translation has become an essential capability. LLMs can assist in translating code from one programming language to another, enabling developers to leverage the strengths of different languages.
This use case is particularly valuable for teams working on cross-platform projects or those migrating legacy systems to modern languages. By automating code translation, LLMs reduce the effort required to rewrite code while ensuring consistency and accuracy.
Example:
Suppose a developer has the following JavaScript function:
javascript
function add(a, b) {
return a + b;
}
An LLM can translate this function to Python seamlessly:
python
def add(a, b):
return a + b
This capability accelerates the development process and facilitates smoother transitions between programming languages.
Learning and Skill Development
LLMs can serve as valuable learning aids for developers seeking to enhance their programming skills. By providing real-time feedback and suggestions, they create an interactive learning environment where developers can experiment with code and receive instant guidance.
New developers can benefit from this use case by asking LLMs specific programming questions or requesting explanations for various programming concepts. This cultivates a more interactive and impactful learning environment.
Example:
A developer might ask an LLM:
"What is the difference between a list and a tuple in Python?"
The LLM could respond with a detailed explanation:
"In Python, a list is a mutable sequence, meaning you can change its content, while a tuple is immutable, meaning once it's created, it cannot be modified. Lists are defined using square brackets [], whereas tuples use parentheses (). Here's an example:
python
my_list = [1, 2, 3]
my_tuple = (1, 2, 3)
This interactive dialogue promotes understanding and retention of programming concepts.
Customized Code Generation
The capability of LLMs to generate customized code snippets tailored to specific requirements is one of their most compelling features. Developers can describe the functionality they need in natural language, and LLMs can translate that description into code, saving time and effort.
This feature is particularly useful for prototyping and brainstorming, where quick iterations are necessary. By enabling rapid code generation, LLMs empower developers to explore multiple solutions to a problem.
Example:
A developer might input:
"Generate a function that fetches user data from an API and returns it as a JSON object."
An LLM can generate:
python
import requests
def fetch_user_data(api_url):
response = requests.get(api_url)
return response.json()
This customized code generation streamlines the development process and encourages creativity in problem-solving.
Enhanced Collaboration
In collaborative environments, LLMs can facilitate smoother communication among team members by providing a shared understanding of code. They can generate explanations, documentation, and comments that bridge the gap between developers with different levels of expertise.
Moreover, LLMs can assist in code reviews by analyzing changes and suggesting improvements. This enhances collaboration by fostering a culture of continuous improvement and knowledge sharing.
Example:
During a code review, a developer might ask an LLM to analyze a pull request:
"What improvements can be made to this code?"
The LLM could respond with insights such as:
"Consider using a more efficient data structure for storing user information. A dictionary might improve lookup times compared to a list."
By providing actionable feedback, LLMs contribute to a more collaborative and effective development process.
Security Enhancement
In today's digital landscape, security is paramount. LLMs can assist developers in identifying potential security vulnerabilities in their code. By analyzing code patterns, LLMs can flag issues such as SQL injection risks or insecure data handling practices.
This proactive approach to security helps developers adopt best practices and enhance the overall safety of their applications.
Example:
If a developer writes code that includes user input without proper validation:
python
query = "SELECT * FROM users WHERE username = '" + username + "'"
An LLM might flag this as a potential SQL injection vulnerability and suggest:
python
import sqlite3
def get_user_data(username):
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
cursor.execute("SELECT * FROM users WHERE username = ?", (username,))
By promoting secure coding practices, LLMs help safeguard applications against common threats.
Integration with Development Environments
LLMs are increasingly being integrated into popular Integrated Development Environments (IDEs) and code editors. This integration allows developers to leverage the power of LLMs directly within their coding environment, streamlining the development process.
With features like code completion, error detection, and documentation generation available at their fingertips, developers can work more efficiently and effectively.
Example:
In an IDE like Visual Studio Code, developers can access LLM-powered extensions that provide real-time code suggestions, automatic documentation, and even contextual explanations. This seamless integration enhances productivity and fosters a more efficient coding workflow.
Conclusion
The integration of Large Language Models in programming assistance and code generation represents a significant leap forward in software development. From intelligent code suggestions to automated documentation, debugging support, and customized code generation, LLMs empower developers to work smarter and more efficiently.
Ready to unlock the potential of your business? Contact Acroplans today to learn more about Empowering Developers with LLM Agents: Revolutionizing Programming Assistance and Code Generation and how it can benefit your organization.
Interested?
Schedule a free consultation, our experts are ready to help you reduce cost and risk while innovating with agility.